'Java investment bank interview' generally contains 'Java Design Pattern' questions. If you want to be a professional Java developer, you should know popular solutions for common\standard coding problems. Such solutions have been proved efficient and effective and always used by experienced developers. These solutions are described as so-called design patterns. Learning design patterns speeds up your experience accumulation in OOA/OOD. Once you grasped them, you would be benefit from them for all your life and jump up yourselves to be a master of designing and developing. Furthermore, you will be able to use these terms to communicate with your fellows more effectively.
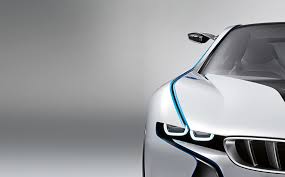
[Details are from taken out from wiki description]
The builder pattern is an object creation software design pattern. The intention is to abstract steps of construction of objects so that different implementations of these steps can construct different representations of objects. Often, the builder pattern is used to build products in accordance with the composite pattern.The intent of the Builder design pattern is to separate the construction of a complex object from its representation. By doing so, the same construction process can create different representations.
Prototype:
Design Pattern - part 2
Many programmers with many years of experience don't know how to use design patterns, but as an Object-Oriented programmer, you have to know them well, especially for new Java programmers. Actually, when you solved a coding problem, you have used one of the design pattern.
You may not use a popular name to describe it or may not choose an effective way to better intellectually control over what you built. Learning how the experienced developers to solve the coding problems and trying to use them in your project are a best way to earn your experience. We have mainly 3 type of design patterns and they are Creational, Structural and Behavioural. Behavioural pattern is explained in next blog - Design Pattern - part 2.
There are frequently asked question in design pattern, and these were asked to me in java interviews:
1) Explain Singleton Design pattern and How to solve Double Check locking?
2) What is Abstract Factory Pattern?
3) What is Adapter pattern?
4) What is Decorator Design Pattern?
5) Where we should use Facade in application?
6) What is Proxy and Composite?
7) What is Observer Pattern?
Creational PatternsThere are frequently asked question in design pattern, and these were asked to me in java interviews:
1) Explain Singleton Design pattern and How to solve Double Check locking?
2) What is Abstract Factory Pattern?
3) What is Adapter pattern?
4) What is Decorator Design Pattern?
5) Where we should use Facade in application?
6) What is Proxy and Composite?
7) What is Observer Pattern?
[Details are from taken out from wiki description]
Factory:
Abstract Factory:
Singleton:
The Singleton pattern is a design pattern that restricts the instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system. The concept is sometimes generalized to systems that operate more efficiently when only one object exists, or that restrict the instantiation to a certain number of objects. The term comes from the mathematical concept of a singleton.
The factory pattern \ factory method pattern is an object-oriented creational design pattern to implement the concept of factories and deals with the problem of creating objects (products) without specifying the exact class of object that will be created. The essence of this pattern is to "Define an interface for creating an object, but let the classes that implement the interface decide which class to instantiate. The Factory method lets a class defer instantiation to subclasses."
Creating an object often requires complex processes not appropriate to include within a composing object. The object's creation may lead to a significant duplication of code, may require information not accessible to the composing object, may not provide a sufficient level of abstraction, or may otherwise not be part of the composing object's concerns. The factory method design pattern handles these problems by defining a separate method for creating the objects, which subclasses can then override to specify the derived type of product that will be created.
The factory pattern relies on inheritance, as object creation is delegated to subclasses that implement the factory method to create objects.
Example : Static factory method provided with Collections class like
Collections.synchronizedMap( new HashMap());
The abstract factory pattern is a software creational design pattern that provides a way to encapsulate a group of individual factories that have a common theme without specifying their concrete classes. In normal usage, the client software creates a concrete implementation of the abstract factory and then uses the generic interfaces to create the concrete objects that are part of the theme. The client does not know (or care) which concrete objects it gets from each of these internal factories, since it uses only the generic interfaces of their products. This pattern separates the details of implementation of a set of objects from their general usage and relies on object composition, as object creation is implemented in methods exposed in the factory interface.
An example of this would be an abstract factory class
DocumentCreator
that provides interfaces to create a number of products (e.g. createLetter()
andcreateResume()
). The system would have any number of derived concrete versions of the DocumentCreator
class like FancyDocumentCreator
or ModernDocumentCreator
, each with a different implementation of createLetter()
and createResume()
that would create a corresponding object like FancyLetter
or ModernResume
. Each of these products is derived from a simple abstract class like Letter
or Resume
of which the client is aware. The client code would get an appropriate instance of the DocumentCreator
and call its factory methods. Each of the resulting objects would be created from the same DocumentCreator
implementation and would share a common theme (they would all be fancy or modern objects).
Example : The best example will be configuring Database connection factory which hide underline Database type. We can configure Oracle, DB2 or Sybase Connection Factory in application.
> java.util.Calendar#getInstance()
> java.util.ResourceBundle#getBundle()
> java.text.NumberFormat#getInstance()
In the second edition of his book Effective Java, Joshua Bloch claims that "a single-element enum type is the best way to implement a singleton" for any Java that supports enums. The use of an enum is very easy to implement and has no drawbacks regarding serializable objects, which have to be circumvented in the other ways.
Builder:
Bill Pugh has written about the code issues underlying the Singleton pattern when implemented in Java. Pugh's efforts on the "Double-checked locking" idiom led to changes in the Java memory model in Java 5 and to what is generally regarded as the standard method to implement Singletons in Java. The technique known as the initialization on demand holder idiom, is as lazy as possible, and works in all known versions of Java. It takes advantage of language guarantees about class initialization, and will therefore work correctly in all Java-compliant compilers and virtual machines.
The nested class is referenced no earlier (and therefore loaded no earlier by the class loader) than the moment that getInstance() is called. Thus, this solution is thread-safe without requiring special language constructs (i.e.
Example: Getting Runtime environment using Runtime class is example of Singleton design pattern --
volatile
or synchronized
). More details on Singleton Pattern.Example: Getting Runtime environment using Runtime class is example of Singleton design pattern --
java.lang.Runtime#getRuntime()
The builder pattern is an object creation software design pattern. The intention is to abstract steps of construction of objects so that different implementations of these steps can construct different representations of objects. Often, the builder pattern is used to build products in accordance with the composite pattern.The intent of the Builder design pattern is to separate the construction of a complex object from its representation. By doing so, the same construction process can create different representations.
- Builder
- Abstract interface for creating objects (product).
- Concrete Builder Provides implementation for Builder. It is an object able to construct other objects. Constructs and assembles parts to build the objects.
Example : Builder pattern is frequently used in Java classes where We need special purpose classes like StingBuilder and StringBuffer.
> java.lang.StringBuilder#append()
> java.lang.StringBuffer#append()
> java.nio.ByteBuffer#put()
Prototype:
The prototype pattern is a creational design pattern used in software development when the type of objects to create is determined by a prototypical instance, which is cloned to produce new objects. This pattern is used to:
- avoid subclasses of an object creator in the client application, like the abstract factory pattern does.
- avoid the inherent cost of creating a new object in the standard way (e.g., using the 'new' keyword) when it is prohibitively expensive for a given application.
To implement the pattern, declare an abstract base class that specifies a pure virtual clone() method. Any class that needs a "polymorphic constructor" capability derives itself from the abstract base class, and implements the clone() operation.
The client, instead of writing code that invokes the "new" operator on a hard-coded class name, calls the clone() method on the prototype, calls a factory method with a parameter designating the particular concrete derived class desired, or invokes the clone() method through some mechanism provided by another design pattern.
Example: Best example will be Java.lang.Object#clone() method. Class has be implement Cloneable interface to use this.
We are covering here -'Java
garbage collection interview questions' or 'Java memory
interview questions' in d...
|
Java util.collections is one of the most important package in java and the important of this package can be understand by...
|
Java Concurrency interview
question - In year 2004 when technology gurus said innovation
in Java is gone down and Sun Microsystems [Now Or...
|
'Java investment bank interview'
generally contains 'Java Design Pattern' questions. If you want
to be a professional Java ...
|
Great stuff! More nice questions and answers right here:
ReplyDeleteDesign pattern questions
Really informative post .
ReplyDeleteComposite design pattern is based on creating a tree structure in such a way that an individual leaf of the tree can be treated just like entire tree composition.
Java composite design pattern
OO design pattern
composite tree design
You can do practice with more java interview questions @ http://skillgun.com
ReplyDeleteThank you for this Information !!
ReplyDeleteLINUX INTERVIEW QUESTIONS
Linux FTP vsftpd Interview Questions
SSH Interview Questions
Apache Interview Questions
Nagios Interview questions
IPTABLES Interview Questions
Ldap Server Interview Questions
LVM Interview questions
Sendmail Server Interview Questions
Read more at Linux Troubleshooting
Great work. I'm a great believer that understanding the context where a Design Pattern is applicable is more important than the actual implementation details. This article gives a good overview. I think this video will also be a good guide. https://www.youtube.com/watch?v=0jjNjXcYmAU
ReplyDeleteGreat and Useful Article.
ReplyDeleteOnline Java Training
Online Java Training India
Java Training
Java Training In Chennai
Java Training Institutes In Chennai
Java360
Very good and interesting article... it is very useful for me.... thanks for sharing your views and ideas..
ReplyDeleteJava Training in Chennai
This comment has been removed by the author.
ReplyDeleteHi There,
ReplyDeleteYour writing shines! There is no room for gibberish here clearly you have explained about Design Pattern in Java. Keep writing!
I bought a Diamond wi-fi adapter that's advertised as being compatible with Linux OS's. Turns out, according to Diamond tech support, it's compatible with tlx but i can upgrade to tlx without going on line with the computer needing the upgrade.
Follow my new blog if you interested in just tag along me in any social media platforms!
Cheers,
Preethi.
Hmm, it seems like your site ate my first comment (it was extremely long) so I guess I’ll just sum it up what I had written and say, I’m thoroughly enjoying your blog. I as well as an aspiring blog writer, but I’m still new to the whole thing. Do you have any recommendations for newbie blog writers? I’d appreciate it.
ReplyDeleteTop 250+AWS Interviews Questions and Answers 2019 [updated]
Learn Amazon Web Services Tutorials 2019 | AWS Tutorial For Beginners
Best AWS Interview questions and answers 2019 | Top 110+AWS Interview Question and Answers 2019
Best and Advanced AWS Training in Bangalore | Amazon Web Services Training in Bangalore
AWS Training in Pune | Best Amazon Web Services Training in Pune
AWS Online Training 2018 | Best Online AWS Certification Course 2018
Attend The Python Training in Bangalore From ExcelR. Practical Python Training in Bangalore Sessions With Assured Placement Support From Experienced Faculty. ExcelR Offers The Python Training in Bangalore.
ReplyDeleteThanks a lot for writting such a great Blog. It's really has lots of insights and valueable informtion. I really appreciate, Now i am waiting for your next Blog!!Machine Learning Course
ReplyDeleteNice Blog, I really happy to read your blog, It is unique, informative and helps to improving my knowledge. Thanks for sharing, KEEP IT UP!!
ReplyDeleteArtificial Intelligence Course
Just the way I have expected. Your website really is interesting. ExcelR Machine Learning Course In Pune
ReplyDeleteI will really appreciate the writer's choice for choosing this excellent article appropriate to my matter.Here is deep description about the article matter which helped me more.
ReplyDeletedata science training in indore
Thanks for the useful information. Keep sharing..
ReplyDeleteMachine Learning training in Pallikranai Chennai
Data science training in Pallikaranai
Python Training in Pallikaranai chennai
Bigdata training in Pallikaranai chennai
Spark with ML training in Pallikaranai chennai
"Very good article with very useful information. Visit our websitedata science training in Hyderabad
ReplyDelete"
Really an awesome blog and useful content. Keep updating more blogs again soon. Thank you.
ReplyDeleteData Science Institute in Hyderabad
360DigiTMG offers the best Data Science Courses on market. Enroll now for a bright future.
ReplyDeletebest data science courses in chennai